Estimation
Proper estimation ensures the system is sized appropriately and can scale as needed. Let’s break down the specific resources and dimensions to consider:
- Storage Estimation:
- Relational DB (Aurora): For transaction records, you need to factor in both the read/write operations and the expected data size. Aurora auto-scales based on the storage and IOPS needed.
- NoSQL DB (DynamoDB): Scale throughput capacity based on the number of read/writes. Enable DynamoDB Auto Scaling for high traffic.
- Networking:
- Consider the outbound and inbound network traffic, especially for communications with external payment providers and banks.
- Use AWS Global Accelerator to improve network performance by routing user traffic to the nearest AWS region for reduced latency.
- Compute Estimation:
- AWS Lambda: Can handle a high number of requests due to its automatic scaling. However, for consistently high traffic like 100,000 TPS, it might be more cost-efficient to switch to Amazon ECS (Elastic Container Service) with Fargate or Amazon EKS (Elastic Kubernetes Service) to avoid the high cost of running functions at that scale.
- CPU & Memory: Use AWS auto-scaling to dynamically allocate CPU and memory resources for microservices that may have high variability in workload (e.g., fraud detection services).
APIs
When designing APIs, you should consider the following key factors:
- Idempotency:
- Payments and refunds should be idempotent to prevent duplicate processing. This can be achieved by requiring clients to send an idempotency key with every request.
- API Throttling:
- Use API Gateway’s built-in rate limiting and throttling to protect the backend from overload during peak traffic. You can also implement request quotas for different types of users (e.g., merchants, admins).
- API Caching:
- Cache frequently accessed data (e.g., payment status checks) using Amazon API Gateway Caching to reduce load on the backend.
- Versioning:
- Plan for multiple versions of APIs to allow for backward compatibility when rolling out new features. Using versioned endpoints like
/v1/payments
ensures smooth transition.
- Plan for multiple versions of APIs to allow for backward compatibility when rolling out new features. Using versioned endpoints like
Databases (Expanded)
In a payment system, data consistency, scalability, and performance are crucial. Let’s expand on the database choices further:
- Aurora PostgreSQL (Relational DB):
- Why: Ensures ACID compliance for financial transactions. It’s essential for handling transactional integrity, ensuring that any payment entry is accurate and cannot be lost during processing or network failure.
- Benefits: It offers high throughput and supports read replicas for scaling read-heavy workloads. Aurora also supports automatic failover, ensuring high availability.
- DynamoDB (NoSQL DB):
- Why: Used for services that require low-latency access and can handle non-relational, schema-less data, such as user sessions, payment status, or metadata.
- Benefits: Scales horizontally to handle thousands of requests per second, with support for features like DAX (DynamoDB Accelerator) for caching, and Streams for event sourcing.
- AWS Timestream (Time-series DB):
- Why: Ideal for storing time-based event data like logs of user interactions, payment event flows, and monitoring metrics.
- Benefits: Optimized for storing trillions of events in a cost-efficient and scalable manner. Useful for analytical queries and monitoring trends.
- Redshift or Athena:
- For data analytics and historical reporting, consider using Amazon Redshift or Amazon Athena to query transaction data stored in S3.
Entity Relationship Diagram (ERD) (Expanded)
In a payment gateway, several entities are interconnected. Here is an expanded ERD with additional details:
- User: Holds basic user details (name, email, payment methods).
- Relationships: Can have many Payment records, and can also request Refunds.
- Payment: Core table storing transaction details like amount, currency, status (initiated, pending, success, failed).
- Relationships: A Payment is linked to a Merchant, has multiple Transaction Logs, and may have a Refund.
- Merchant: Holds merchant-specific data like account details, merchant ID, and bank information.
- Relationships: Each merchant can receive multiple Settlements related to their payments.
- Transaction Log: Tracks each event in the lifecycle of a payment (payment initiated, authorized, completed, etc.).
- Relationships: Belongs to a Payment.
- Refund: Represents refund transactions linked back to the original payment.
- Relationships: Linked to the original Payment.
- Settlement: Tracks the merchant’s aggregated payments and settlements, providing a summary for final bank disbursement.
Class Diagram (Expanded)
In an object-oriented system, the class diagram outlines the primary components of the system.
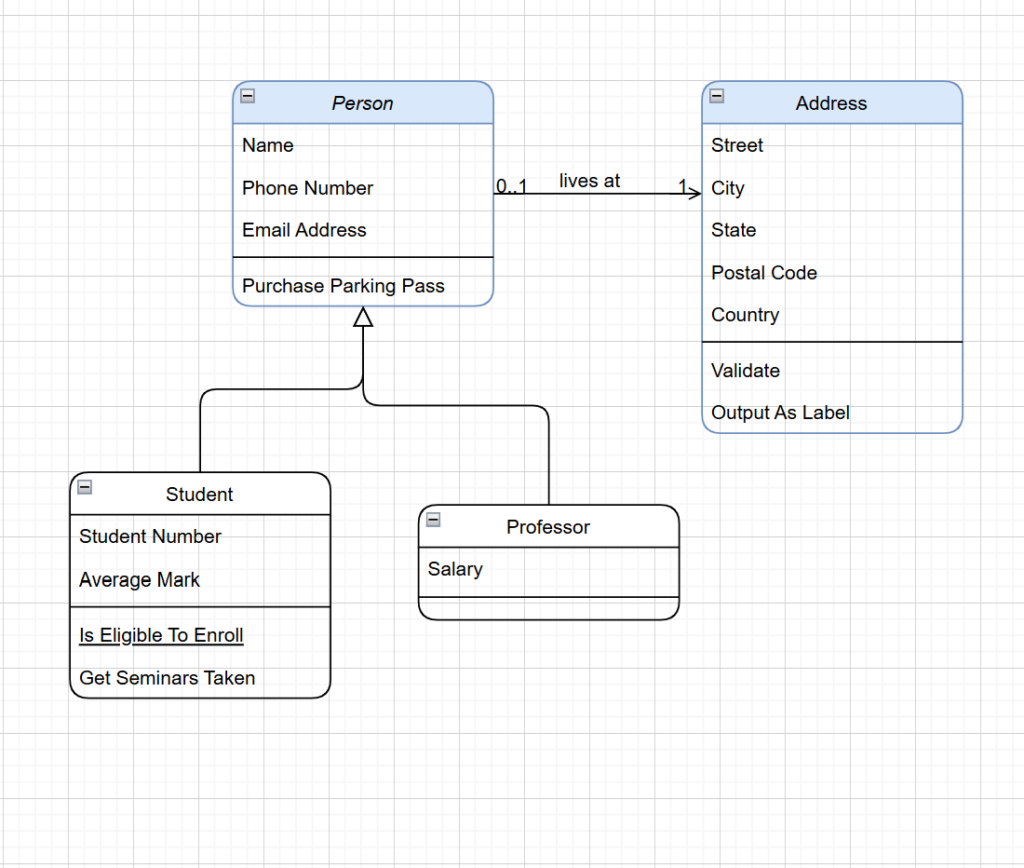
plaintextCopy code+------------------+ +------------------+ +-------------------+
| Payment | | PaymentLog | | Refund |
+------------------+ +------------------+ +-------------------+
| - id: UUID | | - id: UUID | | - id: UUID |
| - amount: Float | | - event: String | | - amount: Float |
| - status: Enum | | - timestamp: Date| | - reason: String |
+------------------+ +------------------+ +-------------------+
^ ^
| |
+-------------------+ +------------------+
| Merchant | | User |
+-------------------+ +------------------+
| - merchantID: UUID| | - userID: UUID |
| - bankInfo: String| | - name: String |
+-------------------+ +------------------+
The Payment class will handle various statuses like initiated, completed, or failed. The PaymentLog class will track various events as the payment flows through the system, including retries, errors, and confirmations.
Sequence Diagram (Expanded)
Here’s an expanded sequence diagram for a successful payment flow:
- User submits a payment.
- API Gateway routes the request to the Payment Service.
- Payment Service:
- Creates a Payment entry in the database (DynamoDB/SQL).
- Publishes an event to the Event Bus (Kafka/SQS).
- Fraud Detection Service (asynchronous):
- Consumes the event and checks for potential fraudulent activity.
- Publishes the result back to the Event Bus.
- Payment Processor:
- Consumes the event and interacts with the External Bank API for authorization.
- Updates the Payment status based on the result (approved or declined).
- Notification Service (asynchronous):
- Sends a success or failure notification to the user and merchant (via Webhooks/Email/SMS).
- Response:
- The Payment Service updates the user on the payment status.
Synchronous and Asynchronous Messaging (Expanded)
- Synchronous:
- Primarily used for interactions where real-time feedback is required, such as when a user initiates a payment and expects a response (e.g., payment initiation status).
- Use HTTP-based APIs with a typical request-response pattern.
- Asynchronous:
- Heavily utilized in event-driven architecture for decoupling services.
- Payment processing involves multiple independent steps (fraud detection, validation, etc.), and these can be processed asynchronously using Amazon SQS/SNS or Kafka.
Example Asynchronous Flow:
- User initiates payment → Payment Service publishes an event to EventBridge.
- Fraud Detection Service consumes the event, processes the transaction, and publishes the result to a new queue.
- Payment Processor consumes the validation event, authorizes the payment, and sends a notification to the merchant asynchronously.
AWS Services (Expanded)
Key AWS services you will leverage:
- Amazon API Gateway: To expose the APIs.
- Amazon SQS/SNS or EventBridge: For event-driven architecture.
- AWS Lambda: For serverless, on-demand execution of small microservices.
- Amazon RDS/Aurora: For transactional data.
- DynamoDB: For high-velocity, non-relational data.
- AWS X-Ray: For tracing distributed transactions.
- CloudWatch: For logs, metrics, and alarms.
- S3: For object storage (e.g., invoices, receipts).
- AWS IAM: To manage permissions across services.
Observability (Expanded)
To ensure proper observability, incorporate:
- Distributed Tracing: Use AWS X-Ray to track payment flows across microservices. This will help detect bottlenecks and improve performance.
- Logging: Implement structured logging using CloudWatch Logs or third-party tools like ElasticSearch.
- Metrics:
- Custom Metrics via CloudWatch (e.g., TPS, payment success/failure rates, API response times).
- Use Prometheus for advanced metrics gathering, and Grafana for visual dashboards.
- Alerting: Set up alerts for critical issues (e.g., increased failure rates or latency) using CloudWatch Alarms or PagerDuty.
Trade-offs (Expanded)
Consistency vs. Availability:
- DynamoDB provides eventual consistency, which works well for most payment status checks but might introduce minor delays in some edge cases. Use strong consistency where needed (e.g., final settlement records).
Cost vs. Performance:
- AWS Lambda is cost-effective at low loads but becomes expensive with heavy, consistent traffic. Transition to ECS/EKS for consistent, high-load traffic.
Monolith vs. Microservices:
- A monolith simplifies the architecture, but scaling becomes harder. A microservices architecture (event-driven) offers better scaling but adds complexity in service coordination, observability, and failure recovery.